Have you ever want to hook your Android App with Youtube API and try to make something cool? I do, and Google’s document kind of sucks, so I am making my own version for people that stumble in the process. Come along!
TL;DR, Source code: YoutubeDataApiDemo
I have simplify the project so the target is to choose a account, login, and get 50 of its’ liked videos.
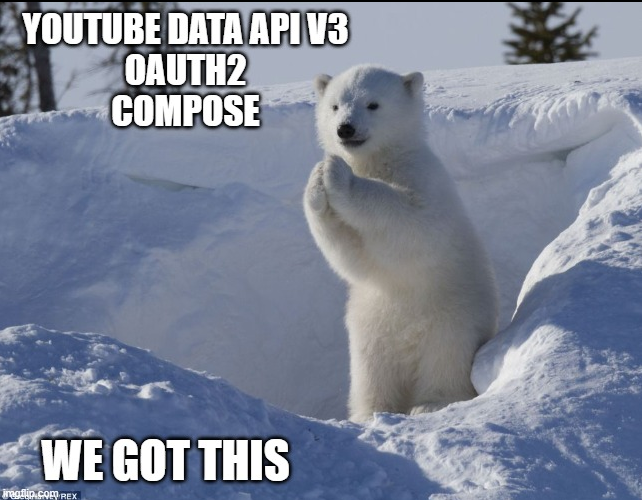
Table of content:
Android Project and library setup
Let start by creating a Empty Android Project, open up your gradle.kts in app and add the following to dependencies
// For viewmodel (You can use hilt)
implementation("androidx.lifecycle:lifecycle-viewmodel-compose:2.8.3")
// Permission
implementation("com.google.accompanist:accompanist-permissions:0.34.0")
// Google play service
implementation ("com.google.android.gms:play-services-auth:21.2.0")
implementation("com.google.api-client:google-api-client-android:2.0.0") {
exclude("org.apache.httpcomponents")
}
implementation("com.google.apis:google-api-services-youtube:v3-rev20240514-2.0.0") {
exclude("org.apache.httpcomponents")
}
after add it, if you are using version catalog, “alt + enter” on each of these implementation to replace it with version catalog declaration, and click sync on the top right.
Next we open AndroidManifest.xml and add the three permission we need above <application> :
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.GET_ACCOUNTS" />
<application
...
Now we will need the package name and SHA1 for our next step. For package name we can find in build.gradle.kts in app, applicationId is what we are looking for.

The SHA1 can be found by clicking the sidebar “Gradle” > open “Tasks” folder > open “android” folder > double click “signingReport”
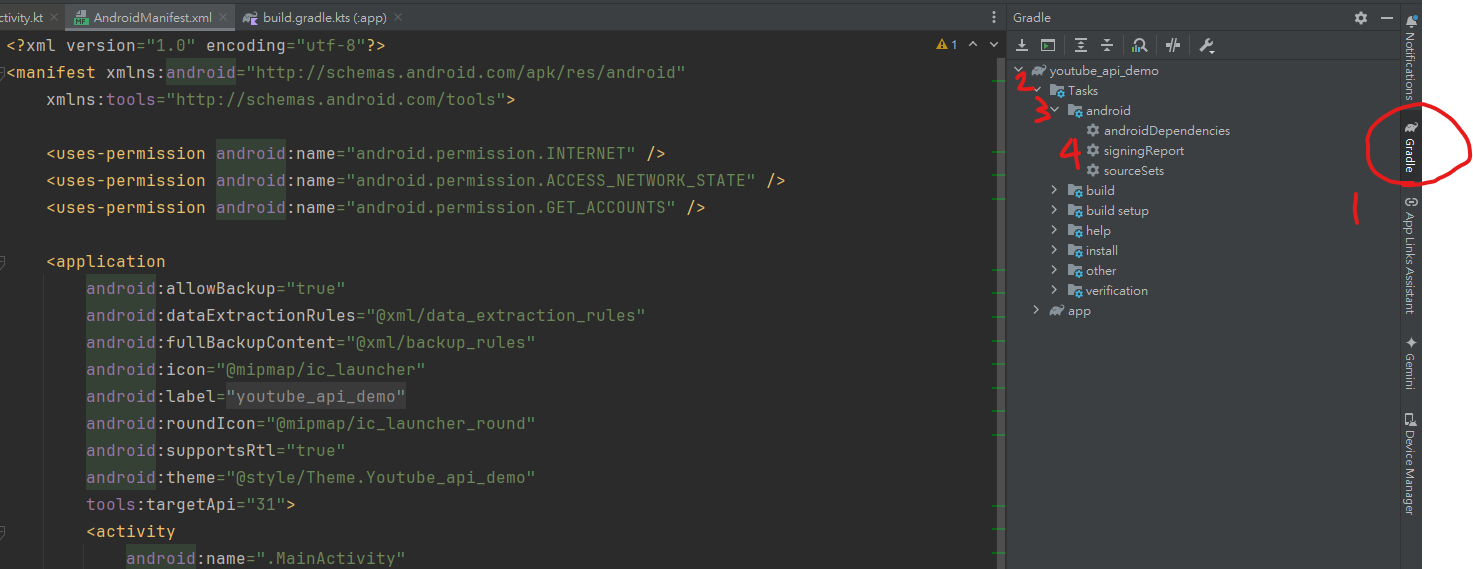
After double click “signingReport”, when finish building, you should be able to see the SHA1 here
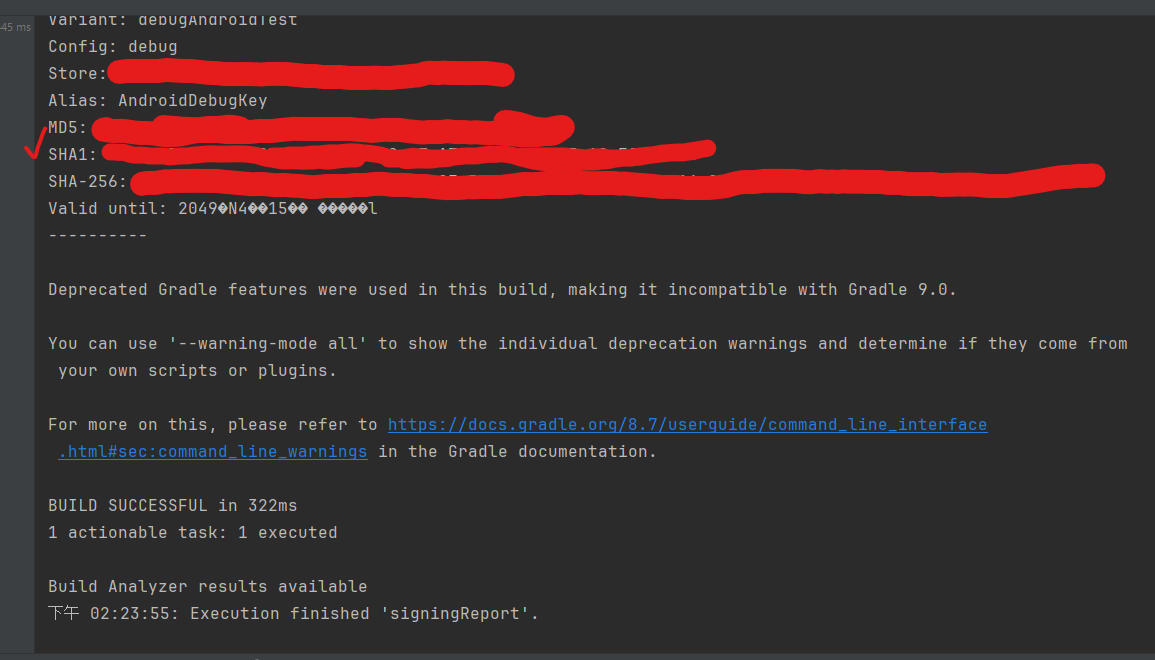
Copy both package name and SHA1 for later usage, now we are set for the next step.
Google Cloud Project setup
Head to Google Cloud and create a project. Then perform the following
A walk through to setup Google Cloud Platform
Now we can start coding.
App coding
I don’t want to make this project to complex, so I will only use a YoutubeScreen.kt for display UI, a LoginViewModel to process login.
We need to know the flow of OAuth login. Here is what we will do.
- Check if google service available.
- Check if we have GET_ACCOUNTS permission, if not, ask for it.
- Prompt user to select an account.
- Try to get credential, if we need consent from user, show it.
- Get the credential token, use Youtube Data Api to get User’s liked video
We do first step in MainActivity, others in YoutubeScreen and YoutubeViewModel.
Sound easy, right?
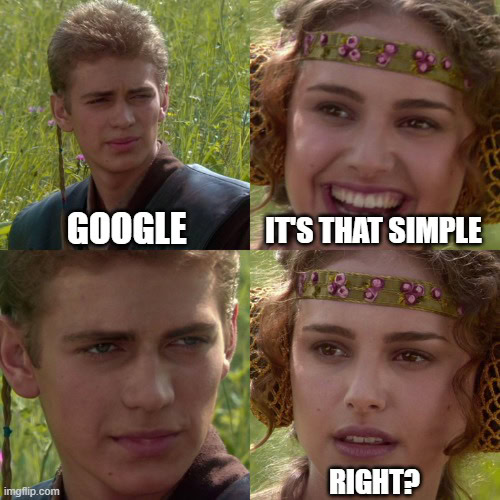
Well, you can try for yourself, but I already done it twice, first time for my App, second time for this post. So let me introduce you our error friends.
Go to This Github project and look for how I set it up.
My Error journey
2 files found with path ‘META-INF/INDEX.LIST’.
In build.gradle.kts(:app), find packaging and edit like following
packaging {
resources {
excludes += "/META-INF/{AL2.0,LGPL2.1}"
excludes += "/META-INF/INDEX.LIST"
}
}
Duplicate class com.google.common.util.concurrent.ListenableFuture found in modules guava-jdk5-17.0.jar
Update your gradle dependecies like following:
// For viewmodel (You can use hilt)
implementation("androidx.lifecycle:lifecycle-viewmodel-compose:2.8.3")
// Permission
implementation("com.google.accompanist:accompanist-permissions:0.34.0")
// Google play service
implementation ("com.google.android.gms:play-services-auth:21.2.0")
implementation("com.google.api-client:google-api-client-android:2.0.0") {
exclude("org.apache.httpcomponents")
}
implementation("com.google.apis:google-api-services-youtube:v3-rev20240514-2.0.0") {
exclude("org.apache.httpcomponents")
}
Permission prompt for contacts not showed
Make sure you add this is AndroidManifest.xml
<uses-permission android:name="android.permission.GET_ACCOUNTS" />
Access Blocked: XXX has not completed the Google verification process
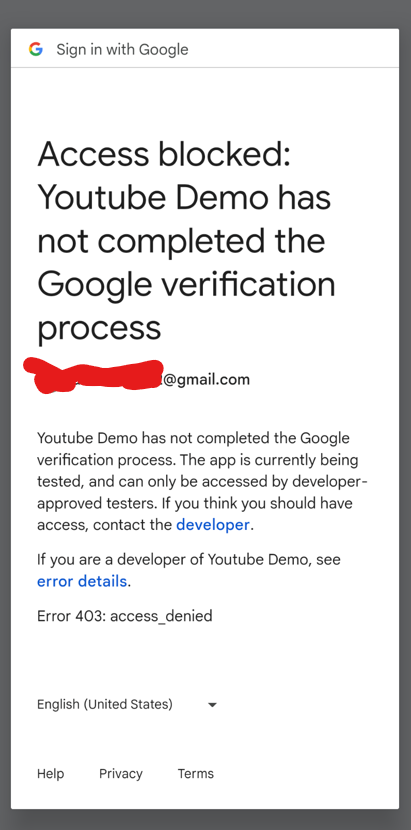
Add this email to Test users in your Google Cloud project.
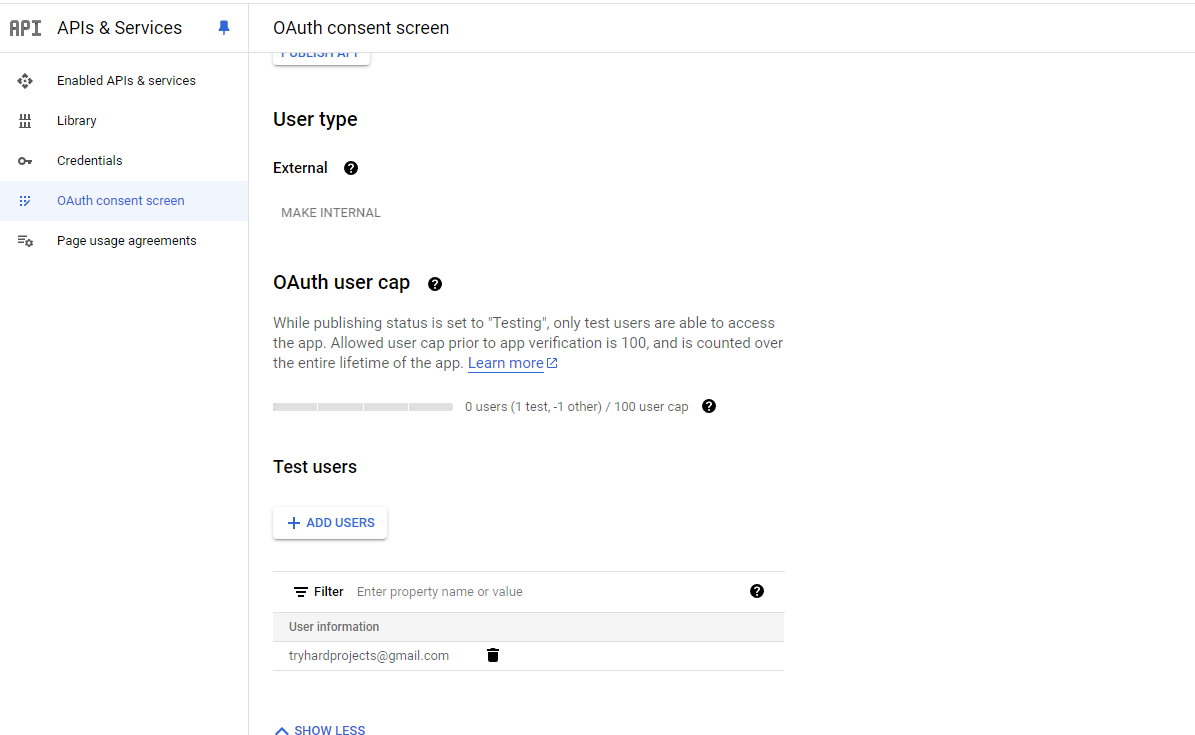
com.google.android.gms.auth.GoogleAuthException: UNREGISTERED_ON_API_CONSOLE
Check your Google Cloud, maybe your package name and SHA1 is wrong. Or check your test user.
Resources
Google’s Youtube Android Guide
API reference so you can test the api online
Conclusion
Although Youtube Data Api is a free api, the limitation quota seems to be too low even for a small project to work on it. You can see for yourself here.
As for playing Youtube video, I tried
android-youtube-player: Very detailed documents and functions, but work better with old Android Views.
YouTubePlayer: A compose based library, easy to use and suit compose very well.
As for me, this is a fun experience, I worked with Youtube api and still trying to figure out what I can do with it, hope this post provide some aid to my fellow tryharders! I will see you next time.